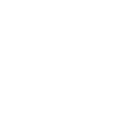
+1
Question regarding the example for Decorator in Python's class-static variable (_component)
Hey there great folk,
Consider the following example for the Decorator pattern in Python: https://refactoring.guru/design-patterns/decorator/python/example
The section that defines the decorator can starts like this:
class Decorator(Component): _component: Component = None def __init__(self, component: Component) -> None: self._component = component @property def component(self) -> Component: return self._component def operation(self) -> str: return self._component.operation()
Is the _component necessary? Can't we just remove it together with the @property method and just initialize it to None inside the __init()__ method?
class Decorator(Component):
def __init__(self, component: Component = None) -> None:
self.component = component
def operation(self) -> str:
return self.component.operation()
Enlighten me.
Customer support service by UserEcho
To be honest, the Python decorator example is just not how it is done in Python at all. This is all you need: